Introduction
Arbitrary delegatecall is a powerful yet potentially risky function in Solidity smart contracts. It can expose systems to vulnerabilities that, if exploited, lead to significant financial loss. This article explains how delegatecall works and details the risks involved when this function is used arbitrarily without proper validation.
What is Delegatecall?
Delegatecall is a special type of function call that allows a contract to execute code from another contract while maintaining its own storage, execution context, and Ether balance. This function allows the caller to:
- Use its own storage layout during execution.
- Run the callee contract’s code as if it were its own.
- Preserve the msg.sender and msg.value from the original function call.
Delegatecall is frequently used in proxy patterns, where a contract separates its storage and logic, with one contract holding data (proxy) and the other handling execution logic (implementation).
How It Works
Delegatecall is especially useful for upgradeable contracts. Here’s how it works in the proxy pattern:
- Proxy Contract: This holds the state variables (storage).
- Logic Contract: This contains the functions and logic that manipulate the proxy contract’s data.
By using delegatecall, the proxy contract can point to different logic contracts over time, making the system upgradeable without altering the stored data.
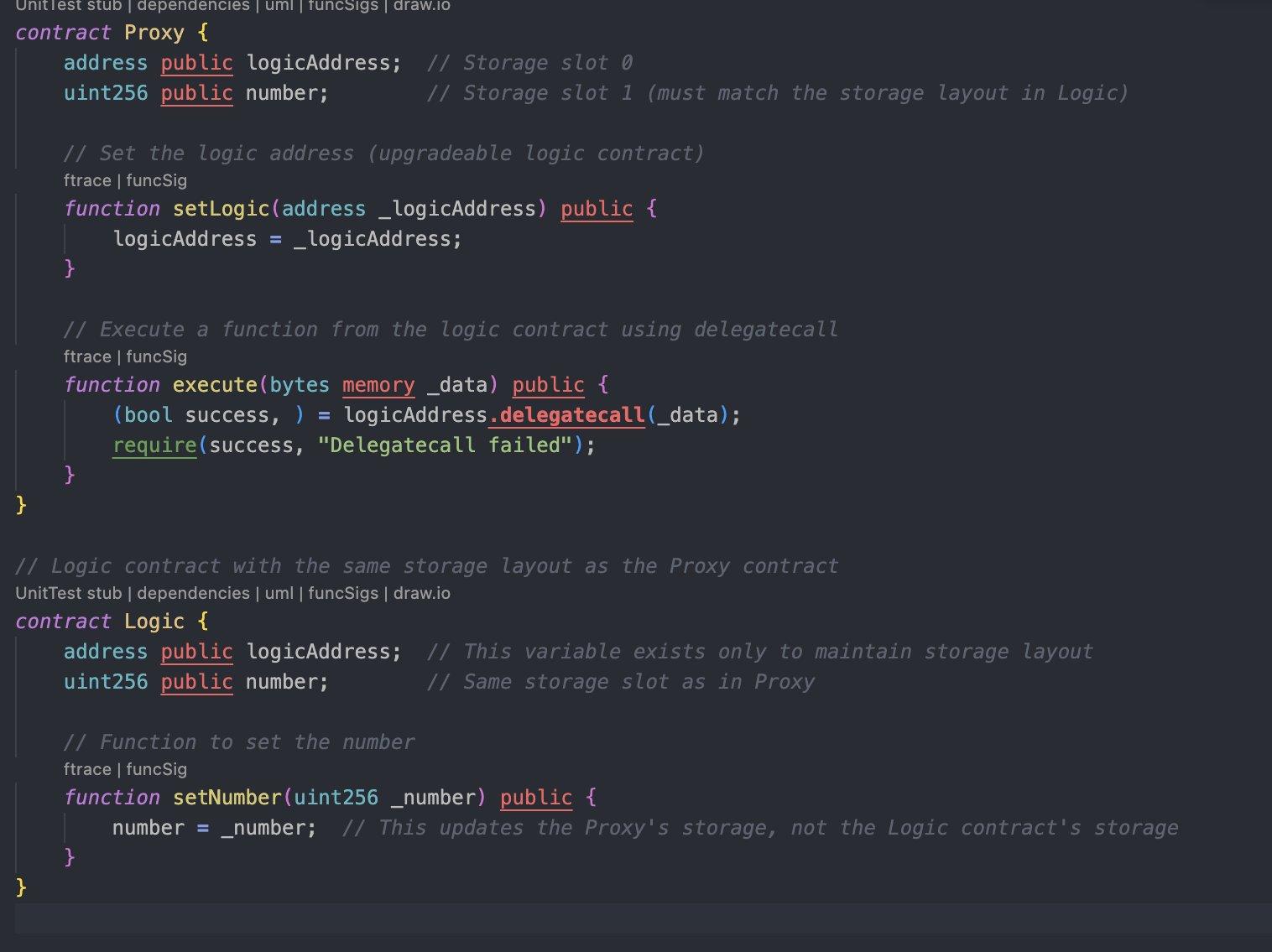
Risks of Arbitrary Delegatecall
Using delegatecall without proper validation—specifically when allowing arbitrary addresses—can lead to serious vulnerabilities. When a contract allows users to specify the target address for delegatecall without thorough checks, an attacker can exploit this by providing a malicious contract’s address.
Potential Consequences:
1. Access to Caller’s Funds: The attacker’s contract can drain funds from the vulnerable contract.
2. Alteration of State Variables: Malicious code can manipulate the caller’s state, leading to unexpected or harmful outcomes.
Attack Scenario
Suppose a contract enables users to pass arbitrary addresses for delegatecall. An attacker could submit the address of a contract specifically designed to execute malicious code, such as transferring funds from the caller contract to the attacker’s address.
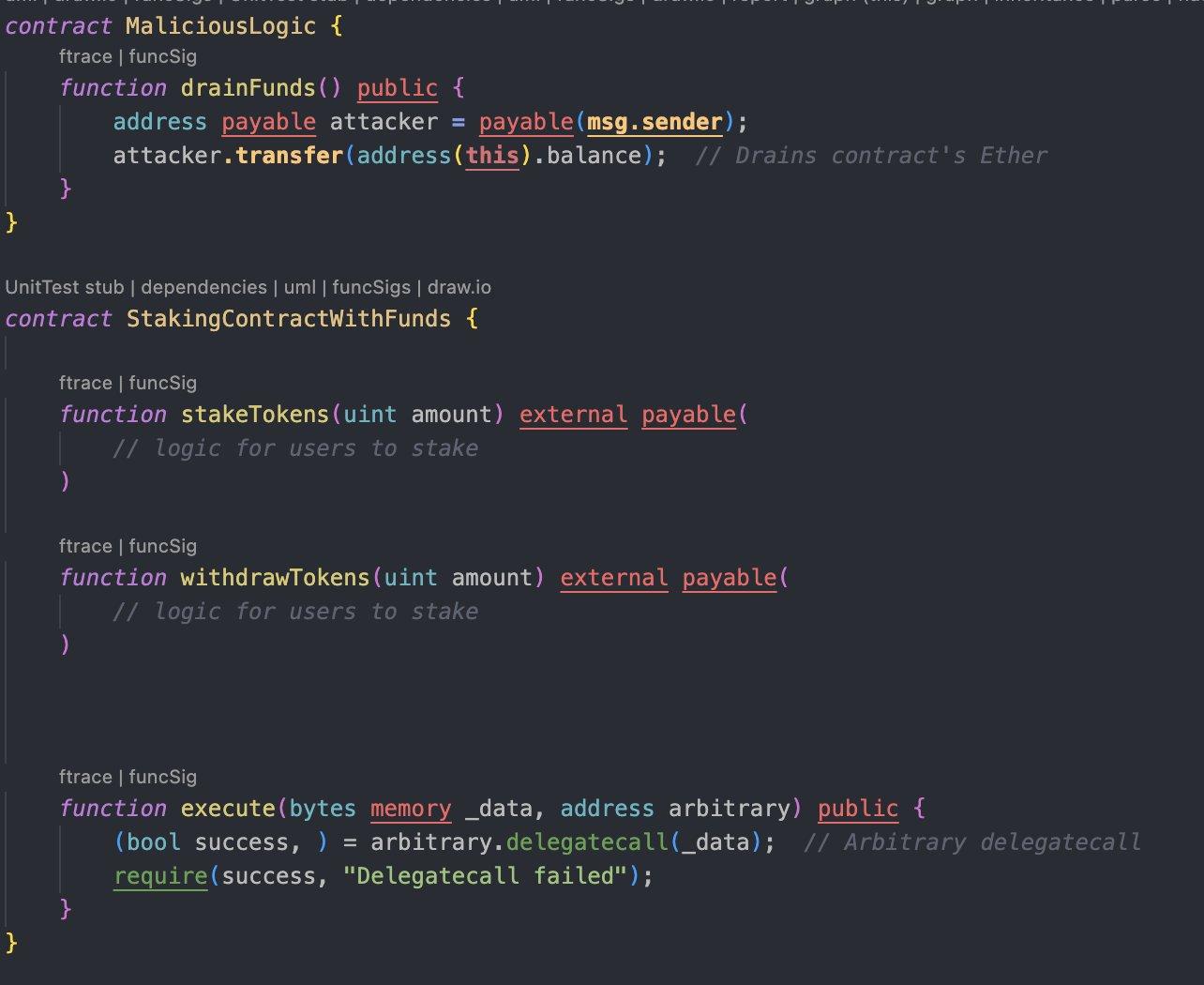
In this case, calling the Malicious contract via delegatecall would allow the attacker to gain control of the caller's execution context, potentially leading to the loss of funds or manipulation of internal state.
Security Tip
Always validate addresses passed to delegatecall to prevent these types of exploits. Contracts should only delegate to trusted, pre-verified logic contracts, and never allow users to specify arbitrary addresses.
Conclusion
Understanding the mechanics and risks of delegatecall is essential for secure smart contract development. While this function provides flexibility and power, improper use—particularly with arbitrary addresses—can lead to vulnerabilities and fund loss. Always ensure that delegatecall is used with proper validation and checks to mitigate these risks.
FAQs
1. What is the main use of delegatecall?
Delegatecall allows a contract to run code from another contract while using its own storage, making it useful for upgradeable contract systems.
2. Why is arbitrary delegatecall risky?
It can allow an attacker to execute malicious code, manipulating the caller's funds and storage, leading to fund theft.
3. How can developers mitigate the risks of delegatecall?
Implement proper validation checks and restrict which addresses can be used for delegatecall to ensure only trusted contracts are executed.
4. What is the difference between call and delegatecall?
The main difference is that delegatecall uses the caller's storage, while call uses the callee's storage.