Introduction
In Ethereum and other blockchain platforms, every transaction has a gas limit, which represents the maximum amount of computational work the transaction can consume. When a function inside a smart contract exceeds this limit, the transaction fails. Loops, particularly when improperly implemented, can lead to gas limit issues, potentially rendering a function uncallable and freezing contract functionality.
The Risk of Loops in Smart Contracts
When smart contracts contain loops, each iteration consumes gas. If there is a way for an attacker or normal user behavior to significantly increase the number of loop iterations, the gas required to execute the function may grow to the point where it exceeds the block gas limit.
This presents a Denial of Service (DoS) vulnerability. When the gas required exceeds the limit, the function cannot be called, causing certain aspects of the contract to become unusable. As a result, critical functions could be frozen, leaving funds or assets inaccessible.
Example of Vulnerable Code
Consider the following example where a contract processes a list of user addresses in a loop:

In this example, the processUsers() function iterates through the entire users array. As the array grows larger, the gas required to complete the loop increases. Eventually, if the array becomes too large, processing all users in one transaction will surpass the block gas limit, resulting in a DoS vulnerability. This could make the function impossible to execute, and dependent operations would also fail.
Fix: Using Batched Loops
A common approach to avoiding this issue is to implement batched operations. Rather than processing all items in a single transaction, the contract can process a fixed number of items per transaction, reducing the gas consumed per operation and ensuring it stays below the block gas limit.
Here’s how you can modify the contract to process users in batches:
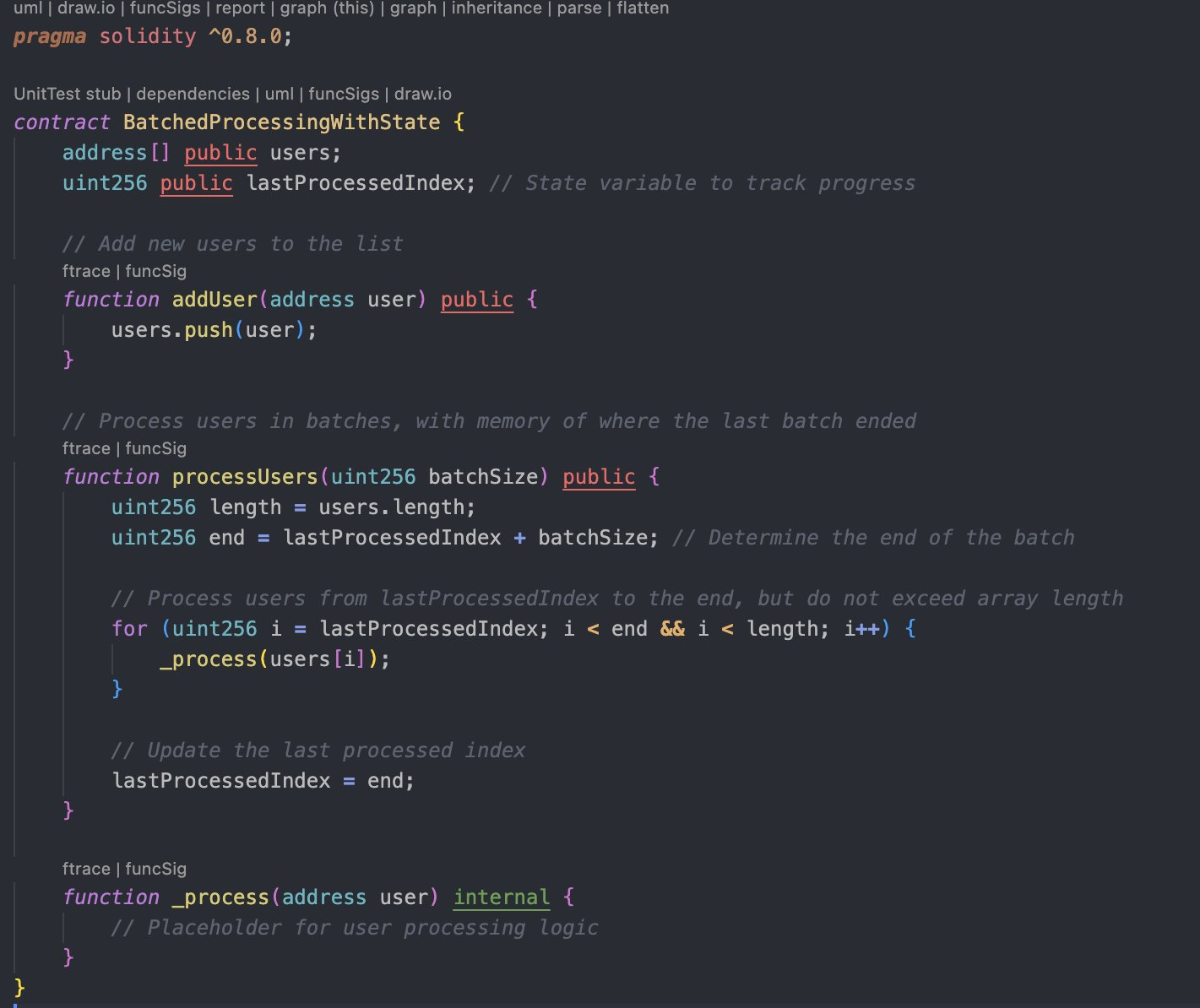
In this updated contract, the processUsersBatch() function processes a batch of users, with each batch limited to a fixed number (batchSize) to ensure gas consumption stays within safe limits. The lastProcessedIndex function keeps track of the last user that was processed, so the next transaction can continue from where the previous one left off.
This method mitigates the risk of exceeding the block gas limit, preventing a DoS vulnerability and allowing the contract to handle large arrays without freezing functionality.
Conclusion
Loops can be risky in smart contracts due to gas limit constraints, particularly when the number of iterations grows unpredictably. Implementing batch processing ensures that no single transaction exceeds the block gas limit, reducing the risk of freezing critical contract functions and improving the scalability of your smart contract.
FAQs
1. What is the block gas limit?
The block gas limit represents the maximum amount of gas all transactions in a block can consume. Exceeding this limit causes a transaction to fail.
2. Why are loops risky in smart contracts?
Loops can become gas-intensive if the number of iterations grows significantly. This may result in exceeding the block gas limit, causing a function to become uncallable.
3. How do batched loops work?
Batched loops process a limited number of items in each transaction, reducing gas consumption and preventing the transaction from exceeding the block gas limit.
4. Can batched loops completely eliminate gas limit issues?
While they can't eliminate gas consumption, batched loops ensure that each transaction consumes a manageable amount of gas, preventing the block gas limit from being exceeded.
5. What happens if the block gas limit is exceeded?
If the block gas limit is exceeded, the transaction fails, and the contract’s function becomes uncallable until the issue is resolved.