Introduction
The `calldatacopy` function in Solidity is a low-level EVM instruction that enables direct copying of data from a transaction's call data into a contract's memory. This operation is particularly useful for contracts that need to handle raw calldata efficiently. This way, it allows developers to access specific parts of the input data without relying on higher-level Solidity functions.
Understanding `calldatacopy`
The `calldatacopy` operation follows this syntax: “calldatacopy(destOffset, calldataOffset, length)”
When executed, `calldatacopy` transfers `length` bytes of data from the specified `calldataOffset` in the transaction's input data to the `destOffset` in the contract's memory. This method is great for handling dynamic calldata. It is very useful for accessing specific function arguments, or optimizing gas usage by bypassing unnecessary high-level data handling.
Example
Consider a scenario where a contract function needs to copy a specific segment of the calldata into memory for processing. Here's how `calldatacopy` can be utilized within an inline assembly block in Solidity:
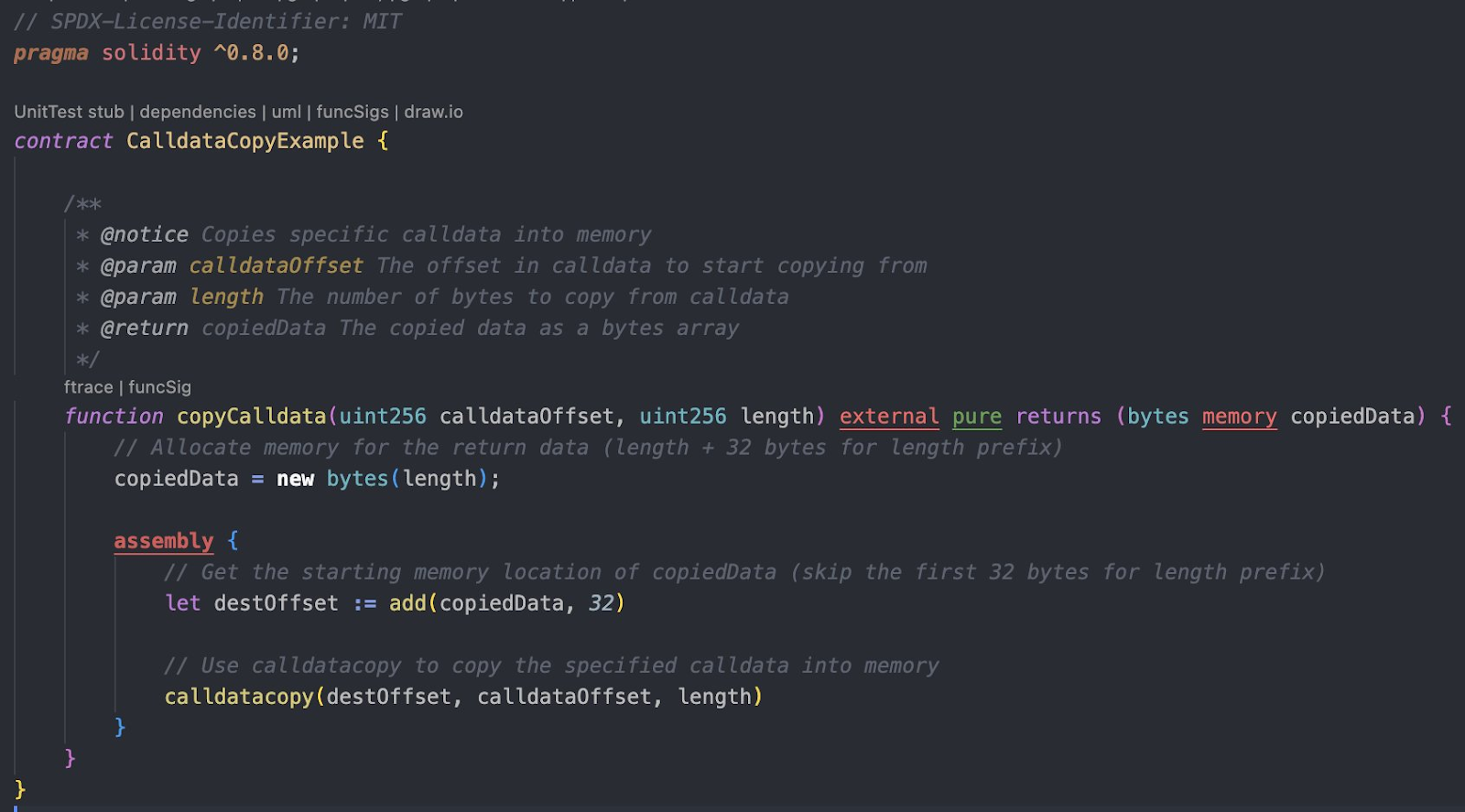
Explanation of the Example
1. Memory Allocation: A dynamic bytes array `copiedData` is created to store the copied segment of calldata. In Solidity, the first 32 bytes of a dynamic array in memory represent its length, so the actual data begins after these 32 bytes.
2. Setting the Destination Offset: Within the inline assembly block, `destOffset` is calculated as `add(copiedData, 0x20)`, which points to the start of the data section in memory, skipping the initial 32 bytes reserved for the array's length.
3. Copying Data Using `calldatacopy`: The `calldatacopy` function copies `length` bytes from the specified `calldataOffset` in the calldata to the `destOffset` in memory, effectively transferring the desired segment of calldata into `copiedData`.
4. Returning the Copied Data: After the data is copied into `copiedData`, the function returns this array, which now contains the specified segment of the original calldata.
By adjusting the `calldataOffset` and `length` parameters, developers can extract specific portions of the calldata as needed, providing flexibility and efficiency in data handling within smart contracts.
For more detailed information on `calldatacopy` and its applications, refer to the Solidity documentation on inline assembly.
Benefits of calldatacopy
for Blockchain Security
Utilizing `calldatacopy` can significantly bolster blockchain security in several ways:
- Direct Data Handling: By managing raw calldata directly, developers can minimize the attack surface associated with higher-level abstractions, reducing potential vulnerabilities.
- Gas Optimization: Efficient gas usage not only lowers transaction costs but also reduces risks associated with gas limit attacks, where an attacker exploits high gas consumption to disrupt contract execution.
- Enhanced Control: Precise manipulation of calldata allows for the implementation of custom security checks and validations, ensuring that only properly structured and authorized data is processed by the contract.
Conclusion
The calldatacopy function in Solidity is a powerful tool for developers, enabling efficient and precise handling of calldata within smart contracts. By allowing direct copying of input data into memory, it facilitates optimized gas usage and enhances contract performance. Moreover, its ability to access specific parts of calldata without relying on higher-level functions contributes to more secure and robust contract development.
FAQs
1. What is the primary purpose of the `calldatacopy` function in Solidity?
The `calldatacopy` function is used to copy a specified number of bytes from the transaction's calldata into the contract's memory. This allows developers to handle input data directly and efficiently within smart contracts.
2. How does `calldatacopy` contribute to gas optimization in smart contracts?
By enabling direct manipulation of calldata, `calldatacopy` reduces the need for higher-level data handling functions, which can be more gas-intensive. This direct approach leads to more efficient gas usage during contract execution.
3. Can improper use of `calldatacopy` introduce security vulnerabilities in a contract?
Yes, if not used carefully, `calldatacopy` can introduce vulnerabilities. For instance, incorrect handling of data offsets or lengths may lead to unintended memory access or data corruption. It's crucial to validate inputs and ensure accurate memory management when using this function.